c Prog to Compute Quotient and Remainder
C Program to Compute Quotient and Remainder
This program evaluates the quotient and remainder when an integer is divided by another integer.
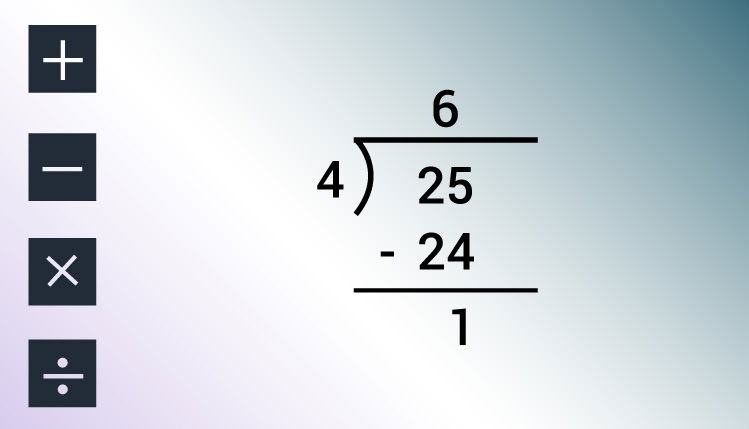
To understand this example, you should have the knowledge of following topics:
Quotient and Remainder
#include <stdio.h>
int main(){
int dividend, divisor, quotient, remainder;
printf("Enter dividend: ");
scanf("%d", ÷nd);
printf("Enter divisor: ");
scanf("%d", &divisor);
// Computes quotient
quotient = dividend / divisor;
// Computes remainder
remainder = dividend % divisor;
printf("Quotient = %d\n", quotient);
printf("Remainder = %d", remainder);
return 0;
}
Output
Enter dividend: 25 Enter divisor: 4 Quotient = 6 Remainder = 1
Comments
Post a Comment